数据集
使用数据集,您可以定义一个测试数据数组,Pest 会自动为每个数据集运行相同的测试。这通过消除手动使用不同数据重复相同测试的需要来节省时间和精力。
1it('has emails', function (string $email) {2 expect($email)->not->toBeEmpty();3})->with(['enunomaduro@gmail.com', 'other@example.com']);
运行测试时,Pest 会自动为使用数据集的测试添加信息丰富的测试描述,概述每个测试中使用的参数,帮助理解数据并在测试失败时识别问题。
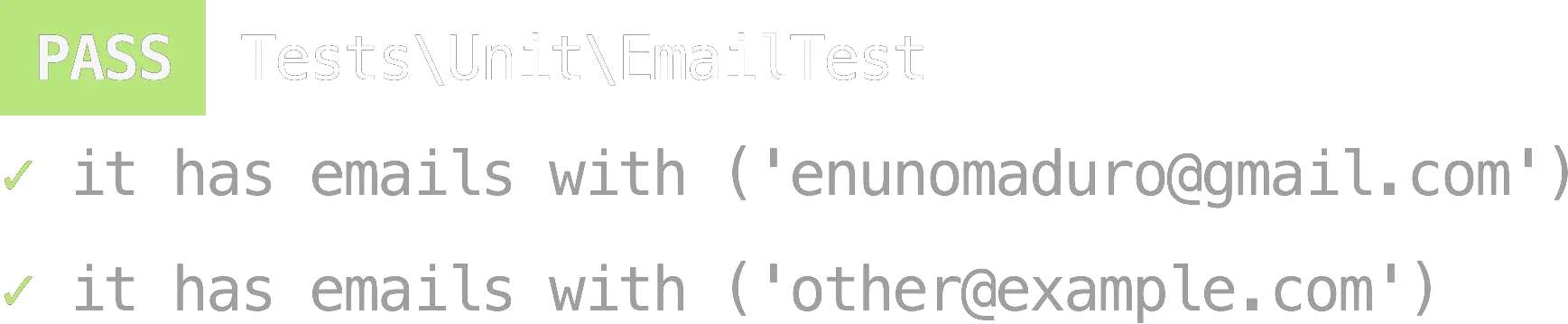
当然,可以通过提供包含参数数组的数组来提供多个参数。
1it('has emails', function (string $name, string $email) {2 expect($email)->not->toBeEmpty();3})->with([4 ['Nuno', 'enunomaduro@gmail.com'],5 ['Other', 'other@example.com']6]);
要手动为数据集值添加自己的描述,只需为其分配一个键即可。
1it('has emails', function (string $email) {2 expect($email)->not->toBeEmpty();3})->with([4 'james' => 'james@laravel.com',5 'taylor' => 'taylor@laravel.com',6]);
如果添加了键,Pest 将在生成测试描述时使用该键。

需要注意的是,在数据集里使用`闭包`时,必须在传递给测试函数的闭包中声明参数类型。
1it('can sum', function (int $a, int $b, int $result) {2 expect(sum($a, $b))->toBe($result);3})->with([4 'positive numbers' => [1, 2, 3],5 'negative numbers' => [-1, -2, -3],6 'using closure' => [fn () => 1, 2, 3],7]);
绑定数据集
Pest 的绑定数据集可用于获取在测试的`beforeEach()`方法之后解析的数据集。这在 Laravel 应用程序(或任何其他 Pest 集成)中特别有用,在这些应用程序中,您可能需要一个`App\Models\User`模型的数据集,这些模型是在`beforeEach()`方法准备数据库模式后创建的。
1it('can generate the full name of a user', function (User $user) {2 expect($user->full_name)->toBe("{$user->first_name} {$user->last_name}");3})->with([4 fn() => User::factory()->create(['first_name' => 'Nuno', 'last_name' => 'Maduro']),5 fn() => User::factory()->create(['first_name' => 'Luke', 'last_name' => 'Downing']),6 fn() => User::factory()->create(['first_name' => 'Freek', 'last_name' => 'Van Der Herten']),7]);
如果需要,可以将单个参数绑定到测试用例。但是,Pest 要求它必须在`it|test`函数参数中完全指定类型。
1-it('can generate the full name of a user', function ($user, $fullName) {2+it('can generate the full name of a user', function (User $user, $fullName) {3 expect($user->full_name)->toBe($fullName);4})->with([5 [fn() => User::factory()->create(['first_name' => 'Nuno', 'last_name' => 'Maduro']), 'Nuno Maduro'],6 [fn() => User::factory()->create(['first_name' => 'Luke', 'last_name' => 'Downing']), 'Luke Downing'],7 [fn() => User::factory()->create(['first_name' => 'Freek', 'last_name' => 'Van Der Herten']), 'Freek Van Der Herten'],8]);
共享数据集
通过将数据集单独存储在`tests/Datasets`文件夹中,可以轻松地将它们与测试代码区分开来,并确保它们不会使主测试文件混乱。
1// tests/Unit/ExampleTest.php... 2it('has emails', function (string $email) { 3 expect($email)->not->toBeEmpty(); 4-})->with(['enunomaduro@gmail.com', 'other@example.com']); 5+})->with('emails'); 6 7// tests/Datasets/Emails.php... 8+dataset('emails', [ 9+ 'enunomaduro@gmail.com',10+ 'other@example.com'11+]);
绑定数据集、描述键以及适用于内联数据集的其他规则也可以应用于共享数据集。
作用域数据集
有时,数据集可能只与特定功能或文件夹集相关。在这种情况下,无需在`Datasets`文件夹中全局分发数据集,而可以在相关文件夹中生成一个`Datasets.php`文件,要求使用数据集并将数据集的作用域限制在该文件夹内。
1// tests/Feature/Products/ExampleTest.php... 2it('has products', function (string $product) { 3 expect($product)->not->toBeEmpty(); 4})->with('products'); 5 6// tests/Feature/Products/Datasets.php... 7dataset('products', [ 8 'egg', 9 'milk'10]);
组合数据集
可以通过组合**内联**和**共享**数据集轻松获得复杂数据集。这样做时,数据集将使用笛卡尔积方法进行组合。
在下面的示例中,我们验证所有指定的企业在提供的每个工作日是否都已关闭。
1dataset('days_of_the_week', [ 2 'Saturday', 3 'Sunday', 4]); 5 6test('business is closed on day', function(string $business, string $day) { 7 expect(new $business)->isClosed($day)->toBeTrue(); 8})->with([ 9 Office::class,10 Bank::class,11 School::class12])->with('days_of_the_week');
运行上面的示例时,Pest 的输出将包含每个验证组合的描述。
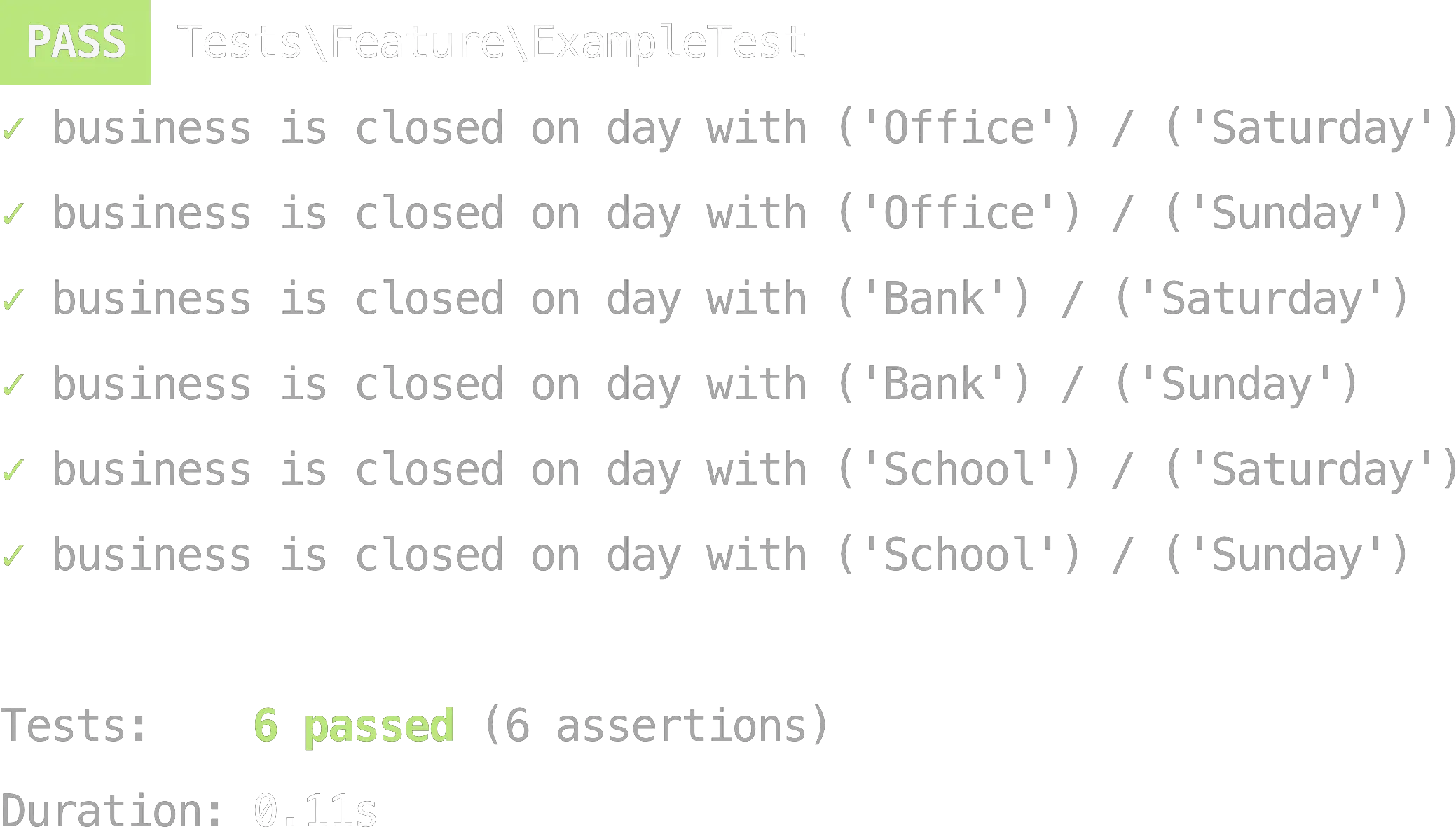
重复测试
在某些情况下,您可能需要重复运行测试多次以进行调试或确保测试稳定。在这些情况下,您可以使用`repeat()`方法重复运行测试指定的次数。
1it('can repeat a test', function () {2 $result = /** Some code that may be unstable */;3 4 expect($result)->toBeTrue();5})->repeat(100); // Repeat the test 100 times
在熟练掌握使用数据集进行测试后,下一步的关键步骤是了解如何测试异常。这包括验证代码在遇到意外或错误输入时是否行为正确并抛出相应的异常:异常 →